3D游戏编程的第五次作业:
- 简单的鼠标打飞碟(Hit UFO)游戏
鼠标打飞碟游戏
游戏要求
游戏内容要求:
- 游戏有 n 个 round,每个 round 都包括10 次 trial;
- 每个 trial 的飞碟的色彩、大小、发射位置、速度、角度、同时出现的个数都可能不同。它们由该 round 的 ruler 控制;
- 每个 trial 的飞碟有随机性,总体难度随 round 上升;
- 鼠标点中得分,得分规则按色彩、大小、速度不同计算,规则可自由设定
游戏要求:
- 使用带缓存的工厂模式管理不同飞碟的生产与回收,该工厂必须是场景单实例的!具体实现见参考资源 Singleton 模板类
- 尽可能使用 MVC 结构实现人机交互与游戏模型分离
游戏实现
游戏实现了:
- 共3个Round,每回合10次Trial;
- 每次Trial中飞碟的颜色、大小、位置,个数等随Round的提升而改变,随机产生但总体难度呈上升趋势;Round 1固定每次只有一个飞碟,后续Round会有几率增加,考虑到同时出现太多点不过来的情况,没有设计同时出现三个以上的情况。
- 不同颜色飞碟的击中得分不同,游戏会记录玩家历次游戏的最高得分;
- 玩家共有10次MISS的机会,超过十次则游戏失败。
游戏设计模式沿用之前的MVC模式,并按照要求使用工厂模式生产飞碟。
3个Round,每回合10次Trial,每次Trial中飞碟的颜色、大小、位置等随Round的提升而改变,总体难度呈上升趋势,不同颜色飞碟不同。
实现过程分析
在本次的游戏设计要求中,对于之前的游戏设计思路又进行了扩展,新增加了工厂模式。具体要求是使用带缓存的工厂模式管理不同飞碟的生产与回收,且工厂必须是场景单实例的。
飞碟工厂用于单独管理预制飞碟的创建和回收,而不再像之前一样由场景控制器管理,使得代码进一步解耦。以下是飞碟工厂的UML图:
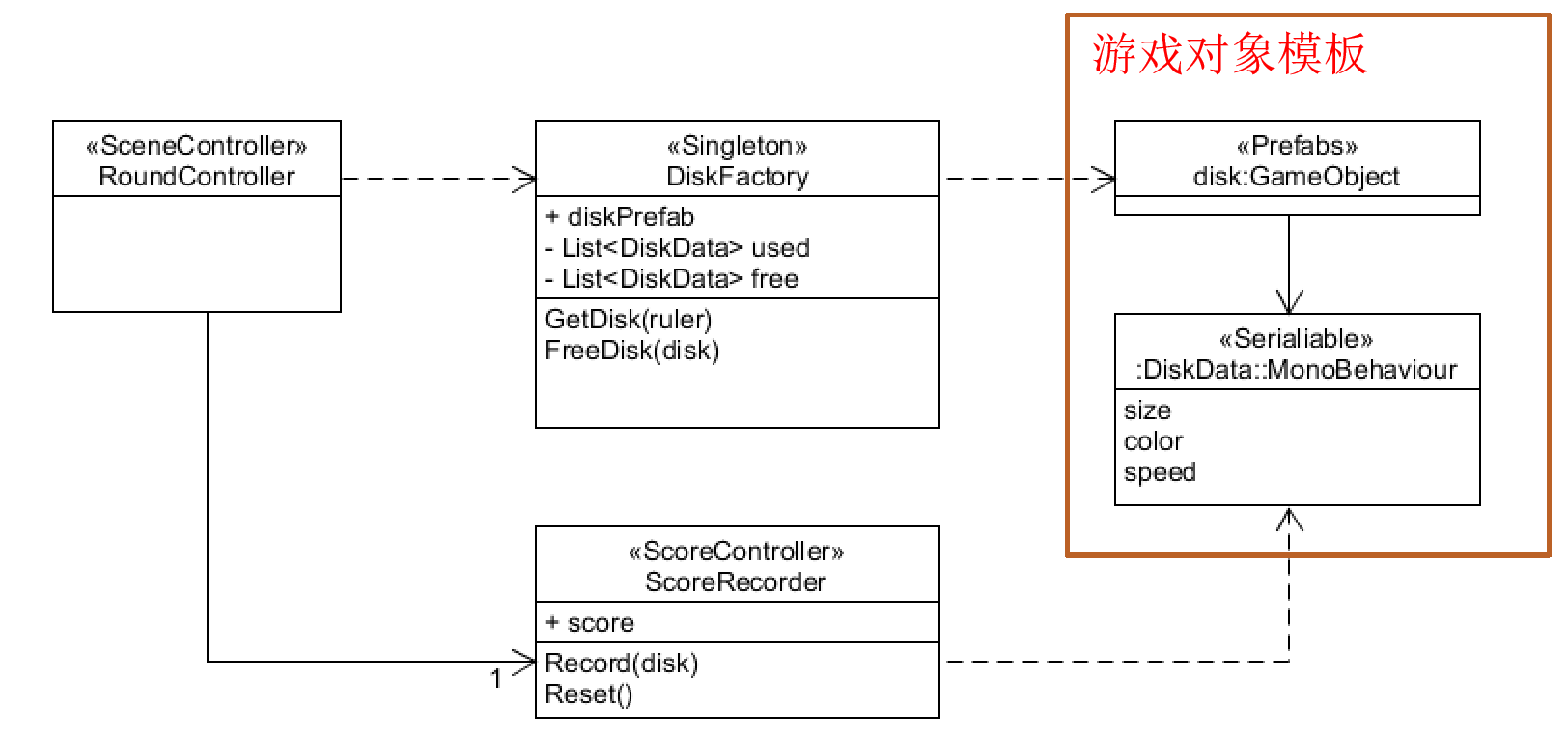
下面介绍一些主要的类,一些基类和接口和之前相同,在这里就不再重复展示了。
飞碟数据类DiskData
1 | using System.Collections; |
飞碟工厂类DiskFactory
带缓存的单实例飞碟工厂主要实现了对于飞碟的创建、管理和回收。他的使用有以下好处:
- 包装了复杂的Disk生产与回收逻辑,易于使用;
- 带缓存的工厂来避免频繁的创建与销毁操作,提高效率:通过两个List实现;
- 包含了Disk产生规则(控制每个round的难度),可以积极应对未来游戏规则的变化,减少维护成本。
1 | public class DiskFactory : MonoBehaviour |
场景控制器 RoundController
场景控制器统筹管理各个组件,管理游戏的整体进程。LoadResource
函数会将当前的回合情况传递给飞碟工厂,以产生相应难度的飞碟。Update
函数每隔一段时间触发一次Trial,即发送飞碟,同时判断是否继续还是结束游戏,并对当前未被击中的飞碟进行回收。此外,还负责处理用户射击事件,判断是否击中并执行后续的行为,如用户扣血,或者分数增加。
1 | public class RoundController : MonoBehaviour, ISceneController, IUserAction |
飞碟动作类CCFlyAction
该类负责实现飞碟的飞行效果,并在下降到一定高度后结束该动作。
1 | public class CCFlyAction : SSAction |
用户交互界面
交互界面比较简单,就是简单的根据游戏的进行状态显示不同的信息,如开始前显示开始游戏,进行时显示当前回合、得分、玩家血量,结束后显示重新开始等字样。
1 | public class UserGUI : MonoBehaviour |
游戏截图
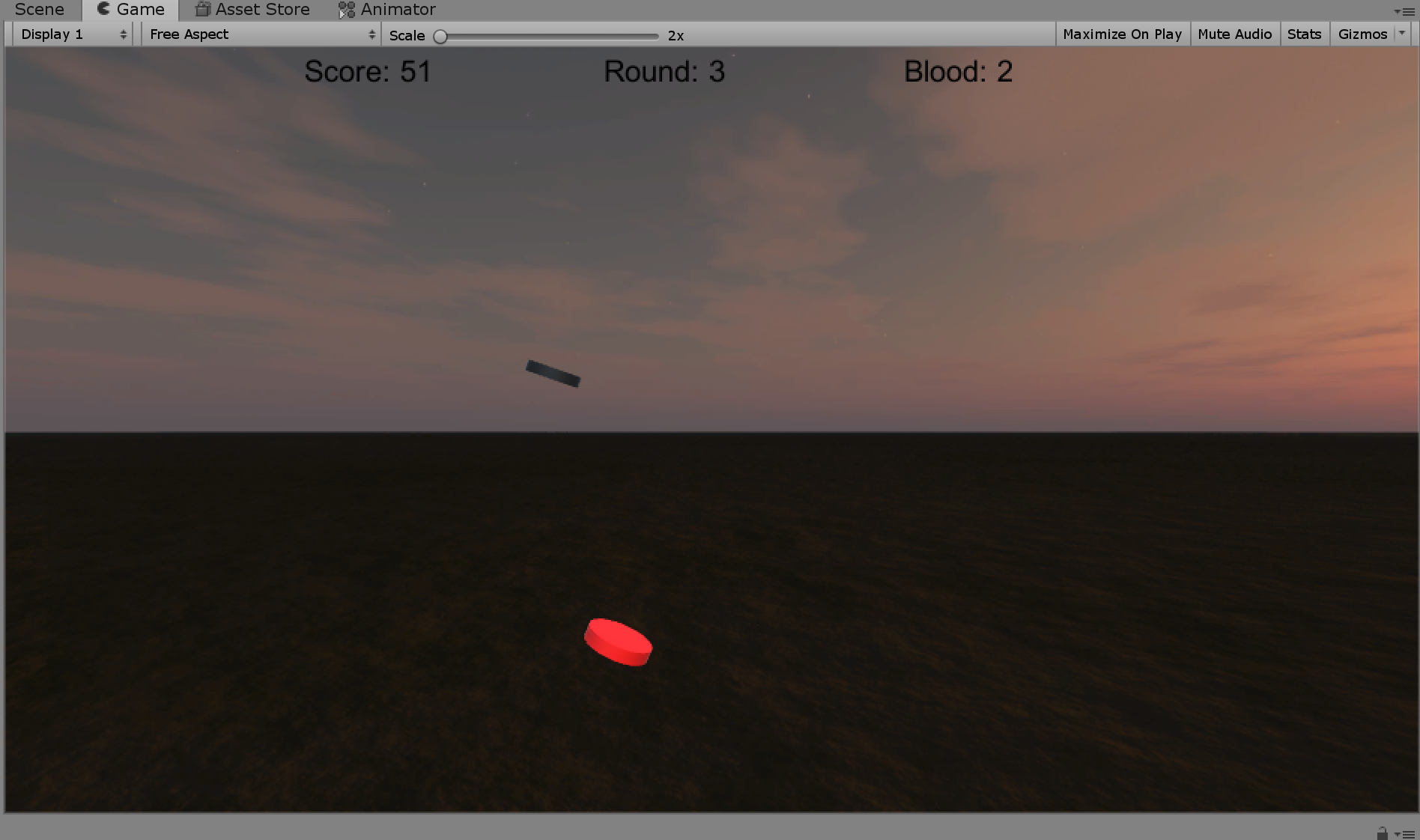
改进空间
- 飞碟重用机制的实现比较粗糙。由于我判断工厂是否需要回收飞碟的方式是:飞碟落回到一定高度下后就回收。这就导致了由于飞碟速度的不同,有时候飞碟回落过慢,导致还未回收,就又需要另外一个飞碟,就会多创建一些新的飞碟出来。
- 用户界面比较粗糙,不够精美。
- 用户射中飞碟后可以添加一些爆炸效果。